A while ago we’ve touched an introduction to UI Library approach where you can see an overview of how you can organize & document user interface components and become more productive in Front-end and Design.
It does not matter what framework you use, but your code should be logically structured following one particular convention and containing only up to date functionality.
Creating CSS module
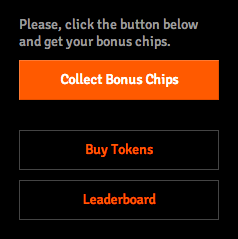
Think component based, not page based. Looking at a design try to analyze every single component whether it’s a button, a checkbox, or a link. Find what’s common between similar components and what’s different. Here is an example with buttons:
We can see 2 different sizes and color skins here. All they have same behavior and font.
Now let’s create a markup for our button and then start styling it with CSS:
/* Button module /stylesheets/modules/button.css */ .button { background-color: transparent; border: 1px solid #444; color: #ff5a00; cursor: pointer; display: inline-block; font-size: 18px; line-height: normal; padding: 8px 15px; position: relative; text-decoration: none; vertical-align: middle; }
Adding module modifier
Then let’s add our orange button as a Modifier
of the base Button Module
:
/* Button module /stylesheets/modules/button.css */
.button {...}
/* Modifier should go below a base module */
.button.is-firm {
background: #ff5a00;
border-color: #fe7a33;
border-bottom-color: #e55000;
color: #fff;
}
Modifier name should always start with prefix is-
(or any prefix you like) in order not to create naming collisions between different modules.
Extending module
Sometimes buttons can have an icon inside. It is called a
Module object
.
By adding a class we allow styling it in the future. Also it is semantically correct and defines the meaning of what the object is about instead of just a span
tag that means nothing until you see how it looks styled.
As an additional benefit you can get out of it is avoiding style inheritance between module objects when adding new ones.
/* Add Button Icon declarations below parent module and its modifiers */
.button-icon {
background: url('images/button/icons.png') no-repeat 0 0;
display: inline-block;
height: 16px;
margin-right: 4px;
vertical-align: middle;
width: 16px;
}
Module object vs Separate module
In the case above, Button icon
can grow pretty fast if you need to add many modifiers to it and they all will become a part of the Button module.
Also, probably, you will need to re-use icons inside other modules (Menu, Tabs and so on), so it’s time to create a new Icon
module.
Move all Button icon related code to a new /stylesheets/modules/icon.css
stylesheet. Don’t forget to update the markup and move icons to an icon folder inside images.
/* Icon module /stylesheets/modules/icon.css */
.icon {
background: url('images/icon/sprite.png') no-repeat 0 0;
display: inline-block;
height: 16px;
margin-right: 4px;
vertical-align: middle;
width: 16px;
}
Thus we reserved a unique name in the system — icon
. Now we can say where exactly the Icon related code is without even seeing a web inspector. Also we know where to find all images related to it.
Hope it will help you to get started with creating your own custom UI components. In the next article, we will touch layouts and utilities for organizing them.