Using Continuous Integration is a must for any mature development process, but in the hurry of delivering to production it’s easy to forget about checking build status before issuing “cap production deploy”. What if while running Capistrano deployment, script will automatically check CI status and prevent us from delivering a broken branch?
In this article, we’ll discuss how this can be easily done via Capistrano recipe. It supports only Travis CI, but can be customised to use with different CI systems.
Implementation
Travis CI provides a gem for their API. This snippet allows us to fetch branch build status:
require 'travis/pro' # this should be pro-token! ci_access_token = "your-pro-access-token" ci_repository = "organisation-or-user/private-repository-name" branch = "master" Travis::Pro.access_token = ci_access_token repo = Travis::Pro::Repository.find(ci_repository) branch_state = repo.branch(branch).state
To access private repository, we need access_token and Pro-account on Travis CI. I hardly imagine somebody deploying from public repository, but anyway, here is how it can be done:
require 'travis' ci_repository = "organisation-or-user/public-repository-name" branch = "master" repo = Travis::Repository.find(ci_repository) branch_state = repo.branch(branch).state
Now we should wrap this snippet into Capistrano recipe and prepare all stuff, like obtaining access to Travis and getting access_token.
Step by step tutorial
0. Prerequisites
You or somebody from your GitHub organization bought Pro-account for Travis CI.
1. Create account in Travis CI
Just login via https://magnum.travis-ci.com/auth with your Github account and grant Travis CI access to your repositories (in case you haven’t done it before).
2. Obtaining access_token
To get access to Pro account API, you need to obtain Travis access_token:
$ gem install travis && travis login --pro && travis token --pro
This command asks your GitHub credentials and outputs access_token:
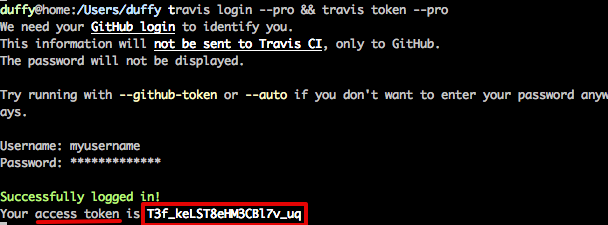
3. Add ‘travis’ gem dependency
Put it into deployment Gemfile:
gem 'travis', require: false
4. Capistrano settings
Put these variables into configuration part of your Capistrano recipes:
set :ci_access_token, "your-pro-access-token" set :ci_repository, "organisation-or-user/repository-name" set :branch do ENV["BRANCH"] || 'master' end
Notice, we need “branch”-variable and this part can differ for your capistrano configuration.
5. Capistrano recipe
Copy this recipe into your recipes directory:
# ci.rb require 'travis/pro' namespace :ci do desc "verification of branch build status on Travis CI" task :verify do begin Travis::Pro.access_token = ci_access_token repo = Travis::Pro::Repository.find(ci_repository) branch_state = repo.branch(branch).state unless branch_state == "passed" Capistrano::CLI.ui.say "Your '#{branch}' branch has '#{branch_state}' state on CI." Capistrano::CLI.ui.ask("Continue anyway? (y/N)") == 'y' or abort end rescue => e Capistrano::CLI.ui.say e.message end end end
6. Enable ci:verify task
Drop this line in front of your deployment steps:
before 'deploy', 'ci:verify'
7. Run deploy
$ cap production deploy
And what we have on the failed build status:
That’s it. Now on every deploy ci:verify will check build status and notify you in case of broken build.